mirror of
https://github.com/FriendsOfTYPO3/feedit.git
synced 2024-11-24 07:06:09 +01:00
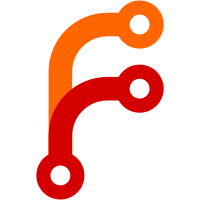
The patch deprecates BackendUserAuthentication->getTSConfigVal() and ->getTSConfigProp() and adapts usages to rely on getTSConfig() without arguments. The array nesting is done directly within consuming code and is combined with ?? to a fallback value. Advantages: * Expensive and recursive string operations within getTSConfig() are not used anymore. * The weird 'value' / 'property' based sub array juggling is gone. * Full TSconfig path including fallback can be easily seen within consuming code * Notice free array access using null coalescence operator Change-Id: I6d5777ebd533dcfdc6018e0226bfb3e513cfa652 Resolves: #84993 Related: #84982 Releases: master Reviewed-on: https://review.typo3.org/56953 Reviewed-by: Anja Leichsenring <aleichsenring@ab-softlab.de> Tested-by: Anja Leichsenring <aleichsenring@ab-softlab.de> Reviewed-by: Stefan Neufeind <typo3.neufeind@speedpartner.de> Tested-by: Stefan Neufeind <typo3.neufeind@speedpartner.de> Tested-by: TYPO3com <no-reply@typo3.com> Reviewed-by: Wouter Wolters <typo3@wouterwolters.nl> Tested-by: Wouter Wolters <typo3@wouterwolters.nl>
71 lines
3 KiB
PHP
71 lines
3 KiB
PHP
<?php
|
|
declare(strict_types = 1);
|
|
|
|
namespace TYPO3\CMS\Feedit\Middleware;
|
|
|
|
/*
|
|
* This file is part of the TYPO3 CMS project.
|
|
*
|
|
* It is free software; you can redistribute it and/or modify it under
|
|
* the terms of the GNU General Public License, either version 2
|
|
* of the License, or any later version.
|
|
*
|
|
* For the full copyright and license information, please read the
|
|
* LICENSE.txt file that was distributed with this source code.
|
|
*
|
|
* The TYPO3 project - inspiring people to share!
|
|
*/
|
|
|
|
use Psr\Http\Message\ResponseInterface;
|
|
use Psr\Http\Message\ServerRequestInterface;
|
|
use Psr\Http\Server\MiddlewareInterface;
|
|
use Psr\Http\Server\RequestHandlerInterface;
|
|
use TYPO3\CMS\Backend\FrontendBackendUserAuthentication;
|
|
use TYPO3\CMS\Core\FrontendEditing\FrontendEditingController;
|
|
use TYPO3\CMS\Core\Utility\GeneralUtility;
|
|
use TYPO3\CMS\Frontend\Controller\TypoScriptFrontendController;
|
|
|
|
/**
|
|
* PSR-15 middleware initializing frontend editing
|
|
*/
|
|
class FrontendEditInitiator implements MiddlewareInterface
|
|
{
|
|
|
|
/**
|
|
* Process an incoming server request and return a response, optionally delegating
|
|
* response creation to a handler.
|
|
*
|
|
* @param ServerRequestInterface $request
|
|
* @param RequestHandlerInterface $handler
|
|
* @return ResponseInterface
|
|
*/
|
|
public function process(ServerRequestInterface $request, RequestHandlerInterface $handler): ResponseInterface
|
|
{
|
|
if (isset($GLOBALS['BE_USER']) && $GLOBALS['BE_USER'] instanceof FrontendBackendUserAuthentication) {
|
|
$config = $GLOBALS['BE_USER']->getTSConfig()['admPanel.'] ?? [];
|
|
$active = (int)$GLOBALS['TSFE']->displayEditIcons === 1 || (int)$GLOBALS['TSFE']->displayFieldEditIcons === 1;
|
|
if ($active && isset($config['enable.'])) {
|
|
foreach ($config['enable.'] as $value) {
|
|
if ($value) {
|
|
if ($GLOBALS['TSFE'] instanceof TypoScriptFrontendController) {
|
|
// Grab the Page TSConfig property that determines which controller to use.
|
|
$pageTSConfig = $GLOBALS['TSFE']->getPagesTSconfig();
|
|
$controllerKey = $pageTSConfig['TSFE.']['frontendEditingController'] ?? 'default';
|
|
} else {
|
|
$controllerKey = 'default';
|
|
}
|
|
$controllerClass = $GLOBALS['TYPO3_CONF_VARS']['SC_OPTIONS']['t3lib/class.t3lib_tsfebeuserauth.php']['frontendEditingController'][$controllerKey];
|
|
if ($controllerClass) {
|
|
$GLOBALS['BE_USER']->frontendEdit = GeneralUtility::makeInstance($controllerClass);
|
|
if ($controllerClass instanceof FrontendEditingController) {
|
|
$GLOBALS['BE_USER']->frontendEdit->initConfigOptions();
|
|
}
|
|
}
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return $handler->handle($request);
|
|
}
|
|
}
|