mirror of
https://github.com/FriendsOfTYPO3/tea.git
synced 2024-12-23 04:06:10 +01:00
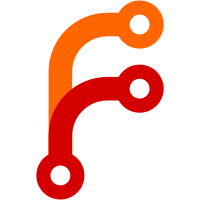
The Prophecy project is basically dead, and our Prophecy dependency currently prevents installations on PHP 8.2 without having to resort to fiddling with Composer's platform options.
94 lines
2.6 KiB
PHP
94 lines
2.6 KiB
PHP
<?php
|
|
|
|
declare(strict_types=1);
|
|
|
|
namespace TTN\Tea\Tests\Unit\Controller;
|
|
|
|
use PHPUnit\Framework\MockObject\MockObject;
|
|
use TTN\Tea\Controller\TeaController;
|
|
use TTN\Tea\Domain\Model\Product\Tea;
|
|
use TTN\Tea\Domain\Repository\Product\TeaRepository;
|
|
use TYPO3\CMS\Core\Http\HtmlResponse;
|
|
use TYPO3\CMS\Extbase\Mvc\Controller\ActionController;
|
|
use TYPO3\CMS\Extbase\Persistence\QueryResultInterface;
|
|
use TYPO3\CMS\Fluid\View\TemplateView;
|
|
use TYPO3\TestingFramework\Core\AccessibleObjectInterface;
|
|
use TYPO3\TestingFramework\Core\Unit\UnitTestCase;
|
|
|
|
/**
|
|
* @covers \TTN\Tea\Controller\TeaController
|
|
*/
|
|
class TeaControllerTest extends UnitTestCase
|
|
{
|
|
/**
|
|
* @var TeaController&MockObject&AccessibleObjectInterface
|
|
*/
|
|
private TeaController $subject;
|
|
|
|
/**
|
|
* @var TemplateView&MockObject
|
|
*/
|
|
private TemplateView $viewMock;
|
|
|
|
/**
|
|
* @var TeaRepository&MockObject
|
|
*/
|
|
private TeaRepository $teaRepositoryMock;
|
|
|
|
protected function setUp(): void
|
|
{
|
|
parent::setUp();
|
|
|
|
// We need to create an accessible mock in order to be able to set the protected `view`.
|
|
$this->subject = $this->getAccessibleMock(
|
|
TeaController::class,
|
|
['forward', 'redirect', 'redirectToUri', 'htmlResponse']
|
|
);
|
|
|
|
$this->viewMock = $this->createMock(TemplateView::class);
|
|
$this->subject->_set('view', $this->viewMock);
|
|
|
|
$this->teaRepositoryMock = $this->getMockBuilder(TeaRepository::class)->disableOriginalConstructor()->getMock();
|
|
$this->subject->injectTeaRepository($this->teaRepositoryMock);
|
|
|
|
$responseMock = $this->createMock(HtmlResponse::class);
|
|
$this->subject->method('htmlResponse')->willReturn($responseMock);
|
|
}
|
|
|
|
/**
|
|
* @test
|
|
*/
|
|
public function isActionController(): void
|
|
{
|
|
self::assertInstanceOf(ActionController::class, $this->subject);
|
|
}
|
|
|
|
/**
|
|
* @test
|
|
*/
|
|
public function indexActionAssignsAllTeaAsTeasToView(): void
|
|
{
|
|
$teas = $this->createMock(QueryResultInterface::class);
|
|
$this->teaRepositoryMock->method('findAll')->willReturn($teas);
|
|
$this->viewMock->expects(self::once())->method('assign')->with('teas', $teas);
|
|
|
|
self::assertInstanceOf(
|
|
HtmlResponse::class,
|
|
$this->subject->indexAction()
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @test
|
|
*/
|
|
public function showActionAssignsPassedTeaAsTeaToView(): void
|
|
{
|
|
$tea = new Tea();
|
|
$this->viewMock->expects(self::once())->method('assign')->with('tea', $tea);
|
|
|
|
self::assertInstanceOf(
|
|
HtmlResponse::class,
|
|
$this->subject->showAction($tea)
|
|
);
|
|
}
|
|
}
|